Ok, you want know if you are a positive or negative person. Or maybe you want to know if your death is close. Or you just want a personality test. Anyway, you are here because you need to build an online test to see results. Here you’ll learn how to write a paged testing system with jQuery.
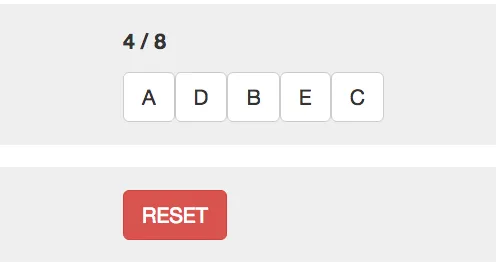
As always, I’ll drop all the code here of a working example for you to read and learn:
index.php
<html lang="en-AU">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>JS Test</title>
<meta name="description" content="Bootstrap basic example by rouralberto">
<meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=no">
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css">
<link rel="stylesheet" href="styles.css">
<script defer src="https://code.jquery.com/jquery-2.1.4.min.js"></script>
</head>
<body>
<?php
$pages = 9;
$i = 1;
$categories = array(
'A',
'B',
'C',
'D',
'E'
); ?>
<script src="main.js"></script>
<script>
var options = <?php echo json_encode($categories); ?>;
</script>
<?php while ( $i <= $pages ) { ?>
<section class="page hide">
<div class="container">
<?php echo '<p><strong>' . $i . ' / ' . $pages . '</strong></p>';
shuffle( $categories );
foreach ( $categories as $category ) {
echo '<button class="save-profile btn btn-default" data-info="' . $category . '">' . $category . '</button>';
} ?>
</div>
</section>
<?php $i ++;
} ?>
<section>
<div class="container">
<button class="reset btn btn-danger">RESET</button>
</div>
</section>
<div class="container results"></div>
</body>
</html>
styles.css
section {
background: #eee;
padding: 15px 0;
margin: 15px 0;
}
main.js
$(function () {
profiler.init();
});
var profiler = {
init: function (e) {
profile = [];
results = [];
$('.results').empty();
$('.page').addClass('hide').first().removeClass('hide');
!e && profiler.events();
},
events: function () {
$('.save-profile').on('click', function () {
profile.push($(this).attr('data-info'));
$(this).parents('section').addClass('hide').next('section').removeClass('hide');
});
$('.page').last().on('click', function () {
$(options).each(function (index, value) {
var occurrences = $.grep(profile, function (element) {
return element === value;
}).length;
results[value] = occurrences;
});
max_value = -1;
for (item in results) {
if (results[item] > max_value) {
max_value = results[item];
result = item;
}
}
$('.results').html('<strong>' + result + '</strong>');
});
$('.reset').on('click', function () {
profiler.init(1);
});
}
};
And thats it! Now you can download the attached ZIP and play!